Last update on
1. Targeted reader
This documentation is intended for users of the MicroSERVER application, allowing the visualization of data supplied from SIREA PLCs.
2. Software presentation
…
3. API
3.1 Obsolescence
This API is available on MicroSERVER until version vX.
3.2 General
This document details the HTTP requests that will allow the user to interact with MicroSERVER for CGI processing, thus providing an interface between the Web server and client programs.
3.3 Functions
3.3.1 Authentification
Python syntax:
import requests
server_uri = 'URI'
user = 'USER'
password = 'PASS'
query = 'QUERY'
resp = requests.post(server_uri + query, {'usr': user, 'pwd': password})
AJAX syntax:
var user = 'USER';
var password = 'PASS';
var query = 'QUERY';
function on_response(data, status, xhr) {
// do something
}
$.post(query, {usr: user, pwd: password}, on_response);
Parameter | Comment |
---|---|
URI | IP address or hostname of the server hosting the database |
USER | User login |
PASSWORD | User password associated to the user login |
QUERY | Query according to PHP functions listed in the following document |
Warning Use POST method for “usr” and “pwd” parameters for security reasons.
Python query exemple:
import requests
server_uri = 'https://192.168.43.108'
user = 'username'
password = 'password'
query = '/get_variables?dev=1'
resp = requests.post(server_uri + query, {'usr': user, 'pwd': password})
AJAX query exemple:
var user = 'username';
var password = 'password';
var query = '/get_variables?dev=1';
function on_response(data, status, xhr) {
// do something
}
$.post(query, {usr: user, pwd: password}, on_response);
3.3.2 get_variables.php
This query retrieves the value of one or several variables.
/get_variables.php?dev=DEV&var1=N1&var2=N2&...&varn=Nn
Parameter | Comment |
---|---|
DEV | ID of the device |
N1, N2, Nn | ID (or address or name) of the variable from which the value is to be obtained. The same request allows to retrieve the value of one or several variables, with no limit |
Information You can use “dev” parameter OR “var” parameters, but only one of them is mandatory.
Syntax of the XML return script:
<update>
<variable>
<timestamp></timestamp>
<rowid></rowid>
<address></address>
<name></name>
<value></value>
<fvalue></fvalue>
<alarm></alarm>
<ack></ack>
</variable>
<variable>
<timestamp></timestamp>
<rowid></rowid>
<address></address>
<name></name>
<value></value>
<fvalue></fvalue>
<alarm></alarm>
<ack></ack>
</variable>
</update>
Tag | Comment |
---|---|
<timestamp> | Moment of the last change in the variable, measured in seconds since the first of September 1970 |
<rowid> | Variable ID index |
<address> | Variable address |
<name> | Variable name |
<value> | Value of the variable, without format |
<fvalue> | Value of the variable, including the format previously defined in MicroSERVER |
<alarm> | 0 when there are no active alarms associated to this variable and 1 if there are any active alarms associated to this variable |
<ack> | 0 when alarm isn’t acknowledged and 1 if alarm is acknowledged |
Query exemple:
https://192.168.43.108/get_variables.php?dev=4&var1=17&var2=18
XML return script exemple:
<update>
<variable>
<timestamp>1415788199.94717</timestamp>
<rowid>17</rowid>
<value>12</value>
<address>%POW</address>
<name>power</name>
<fvalue>12 W</fvalue>
<alarm>0</alarm>
<ack>0</ack>
</variable>
<variable>
<timestamp>1415788167.98588</timestamp>
<rowid>18</rowid>
<address>%POW</address>
<name>power</name>
<value>10</value>
<fvalue>10 A</fvalue>
<alarm>1</alarm>
<ack>0</ack>
</variable>
</update>
3.3.3 get_al.php
This query retrieves all the active alarms in MicroSERVER, or all active alarms associated to a variable or to a device.
/get_al.php?dev=DEV&var=VAR
Parameter | Comment |
---|---|
DEV | ID of the device |
VAR | ID (or address or name) of the variable from which the value is to be obtained. |
Information You can use “dev” parameter OR “var” parameters.
Syntax of the XML return script:
<update>
<entry>
<timestamp></timestamp>
<log_index></log_index>
<var_index></var_index>
<dev_index></dev_index>
<alarm></alarm>
<ack_timestamp></ack_timestamp>
<ack_user></ack_user>
<ack_comment></ack_comment>
</entry>
</update>
Tag | Comment |
---|---|
<timestamp> | Moment of the last change in the variable, measured in seconds since the first of September 1970 |
<log_index> | Entry ID of the log regarding alarm |
<var_index> | ID index of the queried or associated variable |
<dev_index> | Index of the device to which the variable belongs |
<alarm> | Alarm label associated to the active alarm |
<ack_timestamp> | Moment of alarm acknowledgment, if acknowledged; otherwise this field remains empty |
<ack_user> | Identification of the user that has acknowledged the alarm, if acknowledged; otherwise this field remains empty |
<ack_comment> | Comment related to the alarm acknowledgement indicated by the ack_user if acknowledged; otherwise this field remains empty |
Query exemple:
https://192.168.43.108/get_al.php?dev=4&var=10
XML return script exemple:
<update>
<entry>
<timestamp>1415788199.94717</timestamp>
<log_index>7693</log_index>
<var_index>10</var_index>
<dev_index>4</dev_index>
<alarm>Communication default</alarm>
<ack_timestamp></ack_timestamp>
<ack_user></ack_user>
<ack_comment></ack_comment>
</entry>
</update>
3.3.4 get_bg.php
This query retrieves data from a start date and groups them according to the period requested for each variable.
/get_bg.php?time=TIME&period=PERIOD&tz=TZ&var1=N1&var2=N2&...&varn=Nn
Parameter | Comment |
---|---|
TIME | Beginning of the period as timestamp |
PERIOD | Type of period: – 1 = A year with data grouped by month – 2 = A month with data grouped by day – 3 = A day with data grouped by hour – 4 = An hour with data grouped by 5 minutes periods |
TZ | Timezone string, for example “Europe/Paris” |
N1, N2, Nn | ID (or address or name) of the variable from which the value is to be obtained. The same request allows to retrieve the value of one or several variables, with no limit |
Information “var1” parameter is mandatory.
Syntax of the XML return script:
<update>
<entry>
<var_index></var_index>
<timestamp></timestamp>
<value1></value1>
</entry>
</update>
Tag | Comment |
---|---|
<var_index> | ID index of the queried variable |
<timestamp> | Beginning of the period, measured in seconds since the first of September 1970 |
<value1> | Value of the variable, without format |
Query exemple:
https://192.168.43.108/get_bg.php?time=1415788199.94717&period=1&var=10
XML return script exemple:
<update>
<entry>
<var_index>10</var_index>
<timestamp>1415788199.94717</timestamp>
<value1>4</value1>
</entry>
<entry>
<var_index>10</var_index>
<timestamp>1418380199.94717</timestamp>
<value1>4</value1>
</entry>
</update>
Information The correct field name for “value” is <value1> and not <value>.
3.3.5 get_tr.php
This query retrieves a set of values over a defined period for each variable.
/get_tr.php?mintime=MINTIME&maxtime=MAXTIME&np=NP&pval=PVAL&pmult=PMULT&var1=N1&...&varn=Nn
Parameter | Comment |
---|---|
MINTIME | Beginning of the period as timestamp |
MAXTIME | End of the period as timestamp |
NP | Max limit of values to retrieve |
N1, Nn | ID (or address or name) of the variable from which the value is to be obtained. The same request allows to retrieve the value of one or several variables, with no limit |
PVAL, PMULT | Period under the way “pval * pmult” seconds. For exemple, for “4 hours”, pval = 4 et pmult = 3600. |
Information “var1” parameter is mandatory.
Syntax of the XML return script:
<update>
<entry>
<timestamp></timestamp>
<rowid></rowid>
<var_index></var_index>
<value></value>
</entry>
</update>
Tag | Comment |
---|---|
<timestamp> | Timestamp of the point, measured in seconds since the first of September 1970 |
<rowid> | ID of the log entry of the point |
<var_index> | ID index of the queried variable |
<value> | Value of the point, without format |
Query exemple:
https://192.168.43.108/get_tr.php?mintime=1415788199.94717&maxtime=1418380199.94717&np=1&pval=4&pmult=3600&var1=10
XML return script exemple:
<update>
<entry>
<timestamp>1415788199.94717</timestamp>
<rowid>546</rowid>
<var_index>10</var_index>
<value>24</value>
</entry>
<entry>
<timestamp>1418380199.94717</timestamp>
<rowid>547</rowid>
<var_index>10</var_index>
<value>26</value>
</entry>
</update>
3.3.6 get_log.php
This query retrieves a set of events over a defined period for each variable.
/get_log.php?cat=CAT&lastid=LASTID&period=PERIOD&mintime=MINTIME&maxtime=MAXTIME&var1=N1&...&varn=Nn
Parameter | Comment |
---|---|
CAT | Category of the log to retrieve : – 2 = Events – 3 = Alarms – 4 = Values |
LASTID | ID of the log entry of the point from which to retrieve following results |
PERIOD | Period duration in seconds since current date. |
MINTIME | Beginning of the period as timestamp |
MAXTIME | End of the period as timestamp |
N1, Nn | ID (or address or name) of the variable from which the value is to be obtained. The same request allows to retrieve the value of one or several variables, with no limit |
Information “var1” parameter is mandatory and if “period” is provided, “mintime” and “maxtime” are ignored.
Syntax of the XML return script:
<log>
<entry>
<rowid></rowid>
<dev_index></dev_index>
<var_index></var_index>
<var_address></var_address>
<var_name></var_name>
<value></value>
<ack_timestamp></ack_timestamp>
<ack_user></ack_user>
<ack_comment></ack_comment>
<timestamp></timestamp>
</entry>
</log>
Tag | Comment |
---|---|
<rowid> | ID of the log entry of the point |
<dev_index> | ID index of the associated device (if no variable queried) |
<var_index> | ID index of the queried variable |
<var_address> | Address of the associated variable (if no variable queried) |
<var_name> | Name of the associated variable (if no variable queried) |
<value> | Value of the point, without format |
<ack_timestamp> | Timestamp of the acknowledgment, measured in seconds since the first of September 1970 (if “cat” = 3) |
<ack_user> | Username of the user who acknowledged (if “cat” = 3) |
<ack_comment> | Comment of the acknowledgment (if “cat” = 3) |
<timestamp> | Timestamp of the point, measured in seconds since the first of September 1970 |
Query exemple:
https://192.168.43.108/get_log.php?cat=3&lastid=1234&period=60&var1=10
XML return script exemple:
<log>
<entry>
<rowid>1235</rowid>
<var_index>10</var_index>
<value>1</value>
<ack_timestamp>1415788199.94717</ack_timestamp>
<ack_user>admin</ack_user>
<ack_comment>ok</ack_comment>
<timestamp>1415785429</timestamp>
</entry>
</log>
3.3.7 set_variables.php
This query updates one or several variables.
/set_variables.php?var1=N1&...&varn=Nn&value1=V1&...&valuen=Vn
Parameter | Comment |
---|---|
N1, N2 | ID (or address or name) of the variable from which the value is to be obtained. The same request allows to retrieve the value of one or several variables, with no limit |
V1, V2 | Value of the variable regarding his order in the request as “var” parameter. |
Information “var1” parameter and “value1” parameter are mandatory.
Syntax of the XML return script:
<update>
<variable>
<status></status>
<error></error>
</variable>
</update>
Tag | Comment |
---|---|
<status> | “OK” or “ERR” |
<error> | Corresponds to a numeric error code |
Query exemple:
https://192.168.43.108/set_variables.php?var1=10&var2=11&value1=25&value2=27
XML return script exemple:
<update>
<variable>
<status>OK</status>
</variable>
<variable>
<status>ERR</status>
<error>XXX</error>
</variable>
</update>
3.3.8 set_user.php
This query updates the information related to the user logged in.
/set_user.php?new_pwd=NPASS&locale=LOCALE&email=EMAIL&phone_number=PHONE&active_report_filter=ARF&inactive_report_filter=IRF
Parameter | Comment |
---|---|
NPASS | New password to set to the user login |
LOCALE | Regional format as ISO 639-1 code to set to the user login |
Email to set to the user login | |
PHONE | Phone number format to set to the user login |
ARF, IRF | Active or inactive report filter period of the user login. Each period must be separated by a semicolon, and each period is composed of 4 fields: – First field: Weekday (Monday = 1, Sunday = 7) – Second field: Day of month (1 to 31) – Third field: Month (1 to 12) – Fourth field: Hour (0 to 23) Each field must be separated by space. You can put a value, or several separated by a comma, or a star to say “all”. For exemple: “2,5 * * *;7 1 2,10 3” means all Tuesday or Friday of the year AND Sunday 1st on February or October at 3 AM. |
Warning Only ‘fr’, ‘gb’ and ‘us’ ISO 639-1 code are supported in "locale" parameter.
Syntax of the XML return script:
<update>
<variable>
<status></status>
</variable>
</update>
Tag | Comment |
---|---|
<status> | “OK” or “ERR” |
Query exemple:
https://192.168.43.108/set_user.php?new_pwd=newpass&locale=fr&email=contact@exemple.com&phone_number=0600000000&inactive_report_filter=2,5+*+*+*;7+1+2,10+3
Information Spaces must be replaced by “+” or “%20” in HTTP query to be considered as spaces.
XML return script exemple:
<update>
<variable>
<status>OK</status>
</variable>
</update>
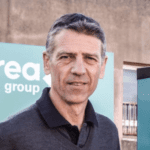